Published
- 2 min read
Working with Lists, Tuples, and Dictionaries in Python
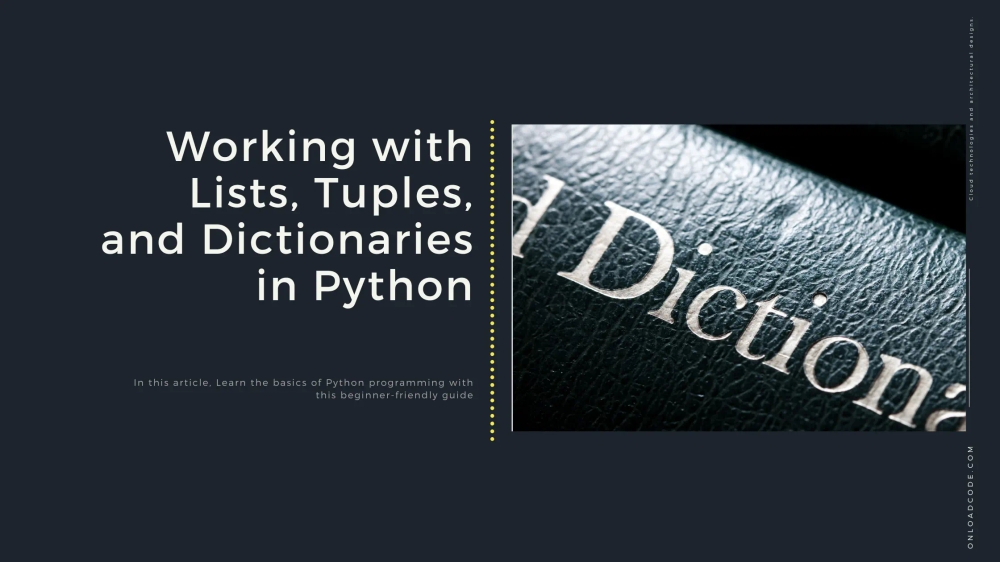
Working with Lists, Tuples, and Dictionaries in Python
Python provides several built-in data structures that help in storing and manipulating data efficiently. This guide covers lists, tuples, and dictionaries.
Lists in Python
A list is an ordered, mutable collection of elements. Lists allow duplicate values and can store different data types.
Creating a List
fruits = ["apple", "banana", "cherry"]
print(fruits)
Accessing Elements
print(fruits[0]) # Output: apple
print(fruits[-1]) # Output: cherry
Modifying Lists
fruits.append("orange") # Adds an element
fruits.remove("banana") # Removes an element
print(fruits)
Looping Through a List
for fruit in fruits:
print(fruit)
Tuples in Python
A tuple is an ordered, immutable collection of elements. Once created, tuples cannot be modified.
Creating a Tuple
colors = ("red", "green", "blue")
print(colors)
Accessing Tuple Elements
print(colors[1]) # Output: green
Tuple Unpacking
r, g, b = colors
print(r, g, b) # Output: red green blue
Dictionaries in Python
A dictionary is an unordered collection of key-value pairs. Each key must be unique.
Creating a Dictionary
person = {"name": "Alice", "age": 25, "city": "New York"}
print(person)
Accessing Values
print(person["name"]) # Output: Alice
Modifying a Dictionary
person["age"] = 26 # Updating a value
person["gender"] = "Female" # Adding a new key-value pair
print(person)
Looping Through a Dictionary
for key, value in person.items():
print(f"{key}: {value}")
Conclusion
Understanding lists, tuples, and dictionaries is fundamental to mastering Python. Lists provide flexibility, tuples ensure immutability, and dictionaries allow fast key-based data retrieval.
Quiz Questions
- What is the main difference between a list and a tuple?
- How do you add an item to a list in Python?
- Can you modify a tuple after creation? Why or why not?
- How do you retrieve a value from a dictionary using a key?
- What method is used to loop through key-value pairs in a dictionary?