Published
- 2 min read
Understanding Variables and Data Types in Python
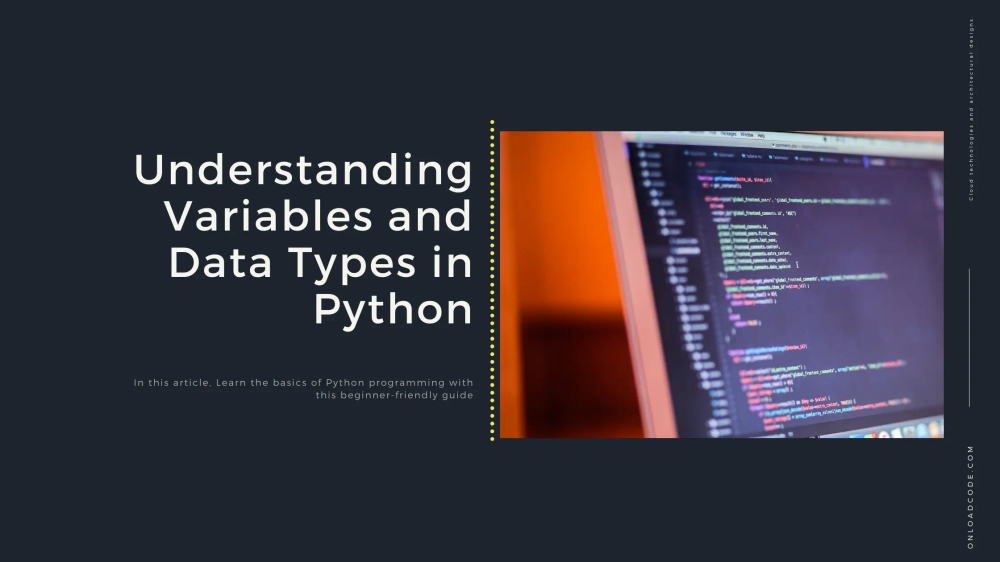
Understanding Variables and Data Types in Python
Variables and data types are foundational concepts in Python programming. This guide will help you understand how to use them effectively.
What is a Variable?
A variable is a container for storing data values. In Python, you don’t need to declare a variable’s type explicitly.
name = "Alice" # String
grade = 85 # Integer
height = 5.7 # Float
is_active = True # Boolean
Python Data Types
Here are some basic data types in Python:
1. Numbers
- Integer: Whole numbers, e.g.,
10
,-5
- Float: Decimal numbers, e.g.,
3.14
,-0.01
age = 30
price = 19.99
2. Strings
- Text enclosed within single or double quotes.
greeting = "Hello, World!"
3. Booleans
- Represents
True
orFalse
values.
is_admin = False
4. Lists
- Ordered, mutable collections of items.
fruits = ["apple", "banana", "cherry"]
5. Tuples
- Ordered, immutable collections of items.
coordinates = (10, 20)
6. Dictionaries
- Unordered collections of key-value pairs.
person = {"name": "Alice", "age": 25}
7. Sets
- Unordered collections of unique items.
unique_numbers = {1, 2, 3}
Dynamic Typing in Python
Python is dynamically typed, meaning you can change a variable’s type by assigning it a new value.
x = 10
x = "Now I'm a string"
print(x)
Type Checking
Use the type()
function to check the data type of a variable.
x = 10
print(type(x))
Type Conversion
Convert between data types using built-in functions:
# Convert float to int
num = int(3.7)
# Convert string to int
num_str = int("10")
# Convert int to string
num_str = str(10)
Best Practices for Naming Variables
- Use meaningful names (
user_age
instead ofua
). - Use lowercase letters and underscores for readability (
user_name
). - Avoid Python reserved keywords (like
class
,def
).
Conclusion
Understanding variables and data types is crucial for effective Python programming. Practice creating different types of variables to get comfortable with these concepts.