Published
- 3 min read
object-oriented-programming— Onload Code
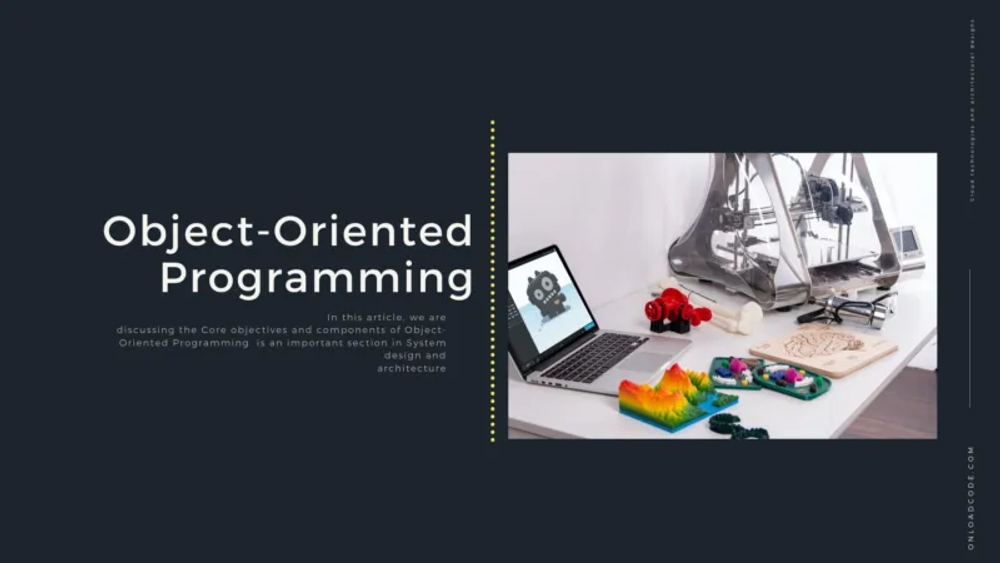
This is the 4th article in the System Design and Software Architecture series.
What is Object-Oriented Programming (OOP)
Object-oriented programming (OOP) is a computer programming (software development) paradigm. It defines the data type of a data structure and the types of operations or functions that can apply to the data structure. In this way, the data structure becomes an object that contains both data and functions. Additionally, programmers can establish connections between one object and another. OOP focuses on the objects developers need to manipulate rather than the logic they need to manipulate.
This programming approach is well-suited for programs that are large, complex, and actively updated or maintained. It is also useful for collaborative development divided into project groups by organizing an object-oriented program.
Characteristics of OOP
Polymorphism
Polymorphism is the ability to efficiently distinguish between OOP programming languages with the same name. Java accomplishes this with the help of method signatures. There are mainly two types of polymorphism in Java:
Runtime Polymorphism (Override)
Runtime polymorphism, also known as dynamic polymorphism or late binding, resolves function calls at runtime.
Example:
@Slf4j
public class Vehicle {
public void tyreCount() {
log.info("How many tires?");
}
}
@Slf4j
public class Car extends Vehicle {
public static void main(String[] args) {
Vehicle vehicle = new Car();
vehicle.tyreCount();
}
@Override
public void tyreCount() {
log.info("4 tyres");
}
}
Output:
4 tyres
Similarly, for a Truck
class:
@Slf4j
public class Truck extends Vehicle {
public static void main(String[] args) {
Vehicle vehicle = new Truck();
vehicle.tyreCount();
}
@Override
public void tyreCount() {
log.info("10 tyres");
}
}
Output:
10 tyres
Compile-time Polymorphism (Overloading)
Compile-time polymorphism, or static polymorphism, is resolved during compilation.
Example:
@Slf4j
public class PrintValues {
public void printValues(int i, int j) {
log.info("{} {}", i, j);
}
public void printValues(int i, int j, int k) {
log.info("{} {} {}", i, j, k);
}
public void printValues(int i, float j) {
log.info("{} {}", i, j);
}
public void printValues(float i, int j) {
log.info("{} {}", i, j);
}
}
Inheritance
Inheritance allows one class to inherit another class’s features or fields and methods, promoting code reuse.
Example:
@Data
public class User {
private String username;
private String firstName;
private String lastName;
}
@Data
@ToString(callSuper = true)
public class AdminUser extends User {
private String organization;
private String department;
}
Encapsulation
Encapsulation wraps data into a single unit, binding code and data together and restricting direct access.
Example:
public class InterestCalculator {
private float principalAmount;
private float interestRate;
private float noOfPeriods;
public InterestCalculator(float principalAmount, float interestRate, float noOfPeriods) {
this.principalAmount = principalAmount;
this.interestRate = interestRate;
this.noOfPeriods = noOfPeriods;
}
public float simpleInterest() {
return (principalAmount * interestRate * noOfPeriods) / 100;
}
}
Abstraction
Abstraction identifies only the required properties of an object while ignoring irrelevant details.
Example:
abstract class Shape {
abstract void getShapeName();
public void whoAmI() {
System.out.println("I'm the Top");
}
}
class Triangle extends Shape {
@Override
void getShapeName() {
System.out.println("Triangle");
}
}
class Rectangle extends Shape {
@Override
void getShapeName() {
System.out.println("Rectangle");
}
}
A Major Advantage of OOP
A significant advantage of object-oriented programming techniques over procedural programming techniques is the ability to create modules that do not require modification when a new type of object is added. Programmers can create new objects that inherit many elements from existing ones, simplifying the process of modifying and extending object-oriented programs.
OOPL — Object-Oriented Programming Languages
Object-oriented programming languages (OOPLs) are high-level languages based on the object-oriented paradigm. Examples include:
- Java
- JavaScript
- Python
- C++
- Visual Basic .NET
- Ruby
- Scala
- PHP
Simula is credited as the first object-oriented programming language.
Conclusion
Thanks for reading the article on Object-Oriented Programming as an essential component in system design and architecture.
Originally published at Onload Code on December 22, 2020.