Published
- 2 min read
Mastering Python Control Flow: If, Else, and Loops Explained
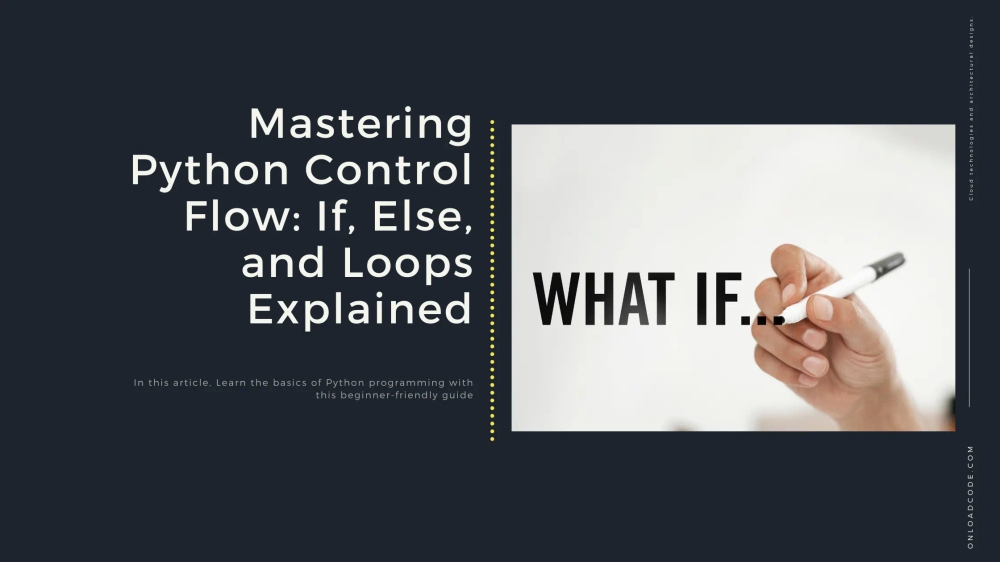
Mastering Python Control Flow: If, Else, and Loops Explained
Control flow allows Python programs to make decisions and repeat actions. This guide covers conditional statements and loops in Python.
Conditional Statements
If Statement
The if
statement allows you to execute code only when a condition is True
.
age = 18
if age >= 18:
print("You are an adult.")
If-Else Statement
Use else
to specify what happens when the condition is False
.
age = 16
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
Elif (Else If)
Use elif
to check multiple conditions.
score = 75
if score >= 90:
print("Grade: A")
elif score >= 80:
print("Grade: B")
elif score >= 70:
print("Grade: C")
else:
print("Grade: F")
Loops in Python
Loops help automate repetitive tasks.
For Loop
Use for
loops to iterate over sequences like lists or strings.
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
While Loop
Use while
loops to repeat a block of code while a condition is True
.
count = 0
while count < 5:
print(count)
count += 1
Break and Continue
break
exits the loop completely.continue
skips the current iteration and moves to the next one.
for num in range(1, 6):
if num == 3:
break # Stops the loop when num is 3
print(num)
for num in range(1, 6):
if num == 3:
continue # Skips 3 and continues
print(num)
Nested Loops
A loop inside another loop is called a nested loop.
for i in range(3):
for j in range(2):
print(f"i: {i}, j: {j}")
Conclusion
Understanding control flow is essential for writing efficient Python programs. Practice using if
, else
, loops, and flow control statements to strengthen your coding skills.
Quiz Questions
- What is the purpose of an
if
statement in Python? - How does the
elif
statement differ fromif
andelse
? - What is the main difference between a
for
loop and awhile
loop? - What does the
break
statement do inside a loop? - How can you skip an iteration in a loop without stopping it completely?