Published
- 3 min read
Java Unit Testing with Nested Tests – Best Practices for Maintainability
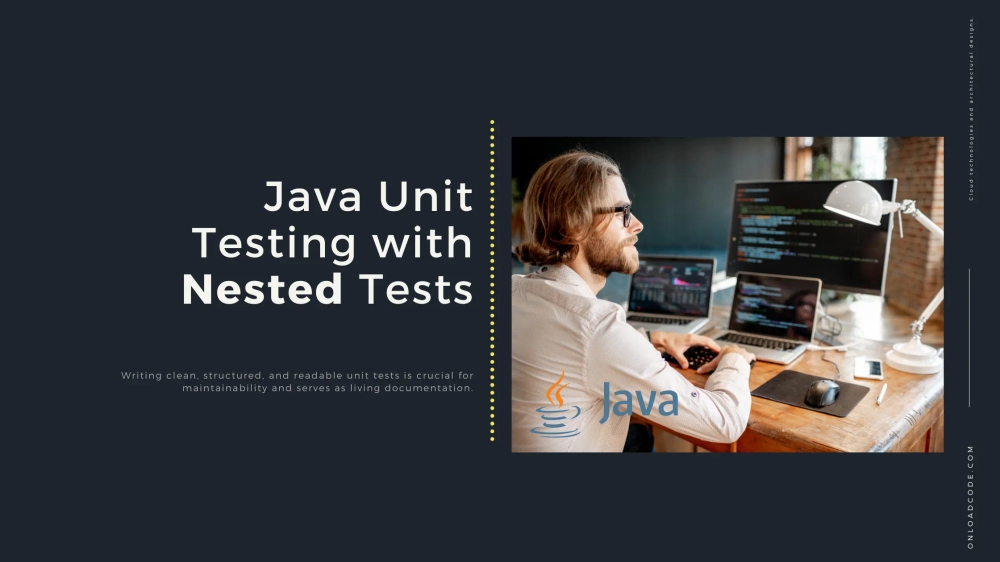
Introduction to Nested Unit Tests in Java
Writing clean, structured, and readable unit tests is as important as writing clean production code. A well-organized test suite not only helps in maintaining a robust codebase but also serves as documentation. JUnit 5 provides the @Nested
annotation, allowing developers to structure related test cases hierarchically, making the tests easier to read and understand.
Why Use Nested Tests?
- Improves readability: Groups related test cases together, making it clear which functionality they test.
- Enhances maintainability: Easier to update or modify specific tests without affecting unrelated ones.
- Reduces code duplication: Shared setup and helper methods keep the test class clean.
- Acts as living documentation: Well-named nested test classes and methods describe system behavior clearly.
Setting Up JUnit 5 for Nested Testing
To use nested tests, ensure you have JUnit 5 (Jupiter API) in your project. Add the following dependency in Maven:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<scope>test</scope>
</dependency>
For Gradle, use:
testImplementation 'org.junit.jupiter:junit-jupiter-api'
Best Practices for Readable and Well-Structured Tests
-
Use Meaningful Test Class Names
- Class names should clearly indicate the functionality being tested.
- Example:
UserServiceTest
instead ofUserTest
.
-
Use
@DisplayName
for Readability@DisplayName
makes test output more descriptive.- Example:
@DisplayName("UserService should correctly validate user input")
-
Follow a Nested Structure for Different Scenarios
- Group related test cases using
@Nested
classes. - Each
@Nested
class represents a specific scenario.
- Group related test cases using
-
Use Given-When-Then Naming Convention
- Clearly define the test scenario in method names.
- Example:
givenValidUsername_whenValidating_thenReturnTrue()
Writing Nested Unit Tests with Meaningful Names (Using Java 17+ Features)
Here’s an example demonstrating best practices for writing readable nested unit tests:
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.*;
@DisplayName("UserService Test Suite")
class UserServiceTest {
private UserService userService;
@BeforeEach
void setup() {
userService = new UserService();
}
@Nested
@DisplayName("User Validation Tests")
class UserValidation {
@Test
@DisplayName("Should return false when username is null")
void givenNullUsername_whenValidating_thenReturnFalse() {
assertFalse(userService.isValidUsername(null));
}
@Test
@DisplayName("Should return false when username is empty")
void givenEmptyUsername_whenValidating_thenReturnFalse() {
assertFalse(userService.isValidUsername(""));
}
@Test
@DisplayName("Should return true for a valid username")
void givenValidUsername_whenValidating_thenReturnTrue() {
assertTrue(userService.isValidUsername("validUser123"));
}
}
@Nested
@DisplayName("User Creation Tests")
class UserCreation {
@Test
@DisplayName("Should throw exception when user data is invalid")
void givenInvalidUserData_whenCreatingUser_thenThrowException() {
assertThrows(IllegalArgumentException.class, () -> userService.createUser(null));
}
@Test
@DisplayName("Should successfully create a user when data is valid")
void givenValidUserData_whenCreatingUser_thenCreateSuccessfully() {
User user = new User("validUser123");
assertDoesNotThrow(() -> userService.createUser(user));
}
}
}
Using Java 17+ Features in Tests
-
Records for Test Data
record User(String username) {}
-
Switch Expressions for Conditional Assertions
String result = switch (userService.getUserStatus("validUser123")) { case "ACTIVE" -> "User is active"; case "INACTIVE" -> "User is inactive"; default -> "Unknown status"; }; assertEquals("User is active", result);
Organizing Nested Tests for Different Scenarios
- Keep a clear separation: Each nested class should focus on a single aspect of the system (e.g., validation, creation, deletion).
- Avoid deep nesting: Two levels are usually sufficient; more than that may reduce readability.
- Use
@BeforeEach
wisely: Avoid unnecessary setup in parent classes that nested classes don’t need.
Conclusion: Making Tests Readable and Maintainable
By following nested unit test best practices, you create a structured, readable, and maintainable test suite. Treat your test cases like documentation—clear method names, @DisplayName
annotations, and properly grouped scenarios make debugging and maintaining tests effortless.
Start using JUnit 5 Nested Tests today and transform your test suite into a self-documenting, high-quality validation tool for your Java applications! 🚀
📖 Read all my articles on Medium: @madukajayawardana.