Published
- 3 min read
How to write explicit code? — Onload Code
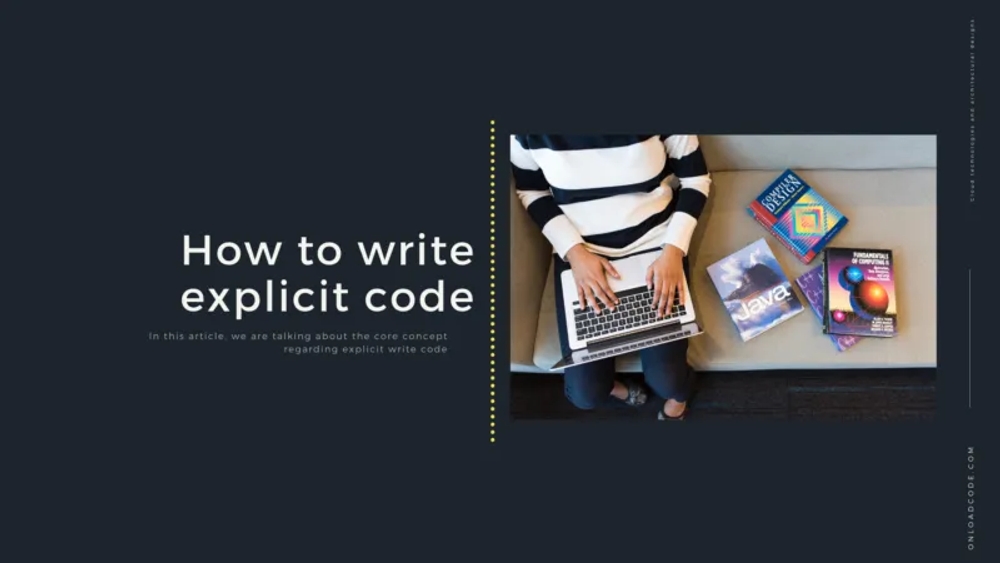
Introduction
You want to know what explicit code is to become an explicit programmer, don’t you? That is why you are reading this article. Unfortunately, you cannot find the exact definition for your question anywhere because it is difficult for us to say for sure what explicit code is like. In simple words, we can say this:
“Clear code is code that is easy to understand, easy to change, and performs fast.”
OK. Let’s dive deeper into this statement.
You are not the only one who reads the code you write. Your colleagues, tech lead, project manager, or a developer who will handle the project after you may read your code many times. When you or they read the code, it should be easy to read and understand. If someone takes less time to read and understand its logic and functionality, it will be easy to understand code. Moreover, easy-to-understand code is less prone to guesswork and misunderstandings. Explicit code is easy to understand in all aspects.
Easy to Understand
- The flow of execution of the whole program
- The way various objects cooperate with each other
- The role and responsibility of each class
- What each function or method does
- The purpose of each variable and expression
Key Practices for Writing Explicit Code
- Well-named classes, methods, and variables: Comply with proper naming conventions and meaningfulness.
- Boolean variables: Use
is_
,has_
, orshould_
prefix, e.g.,$is_valid=FALSE;
- Numeric variables: Names that denote it is numeric, e.g.,
$total_comments=50;
- List variables: Use plural names, e.g.,
$new_names=array(….);
- Method names: Describe functionality, e.g.,
format_comments()
- Boolean variables: Use
- Comments: Describe the purpose of each variable, method, and class.
- Properly structured code: Use proper indentation.
- Compliance with coding standards and conventions.
- Consistency in coding style:
- Placement of braces
- Consistent naming
- Well encapsulated code: Wrap variables and methods together as one unit.
- Well-organized code: Data and functionalities in a class fit together with no extraneous dependencies among classes.
- Separation of concerns: Divide code into multiple chunks designed to perform one specific function.
- Simplicity: Not writing ten lines of code for something that can be done in five.
- Straightforwardness: Do tasks directly without being overly clever.
- Modularity: Divide the source code into multiple independent functional reusable units.
- Elegance: Less complexity in the code.
- Minimum redundancy: Fewer unnecessary codes like dead codes.
- Layer separation: UI does not mix with business logic.
- Properly structured README file: Briefly describe the code’s overview, purpose, approach, metrics, naming conventions, libraries, packages, software license, software version, and author details.
Easy to Change
Another attribute of explicit code is that it can be easily changed. Your code should be easy to extend, refactor, and fix bugs. If the developer making changes can thoroughly understand the code and is confident that the changes will not break functionality, this can be easily achieved. An easy-to-change code has the following properties:
- Use small classes and methods.
- Each class and method complies with the Single Responsibility Principle (SRP).
- Classes with concise public APIs.
- Classes and methods work as expected.
- Easily testable code.
- Easy-to-understand and easy-to-change test cases.
Efficient Performance
Explicit code performs efficiently, avoiding useless costly operations. For example, code that executes costly operations multiple times or triggers N+1 database queries is not clear.
Conclusion
We hope this guide helps you understand what explicit code is. If your colleagues understand your code easily and quickly, change it without breaking functionality, and enjoy working on it, you are writing explicit code.
If you have any other tips on writing explicit code or any related issues, feel free to mention them in the comments section. Don’t forget to share this article to spread the knowledge. Let’s end with a quote:
“Any fool can write code that a computer can understand. Clear programmers write code that humans can understand.” — Martin Fowler
Originally published at https://onloadcode.com on December 8, 2020.