Published
- 2 min read
Handling Errors in Python: Try, Except, and Best Practices
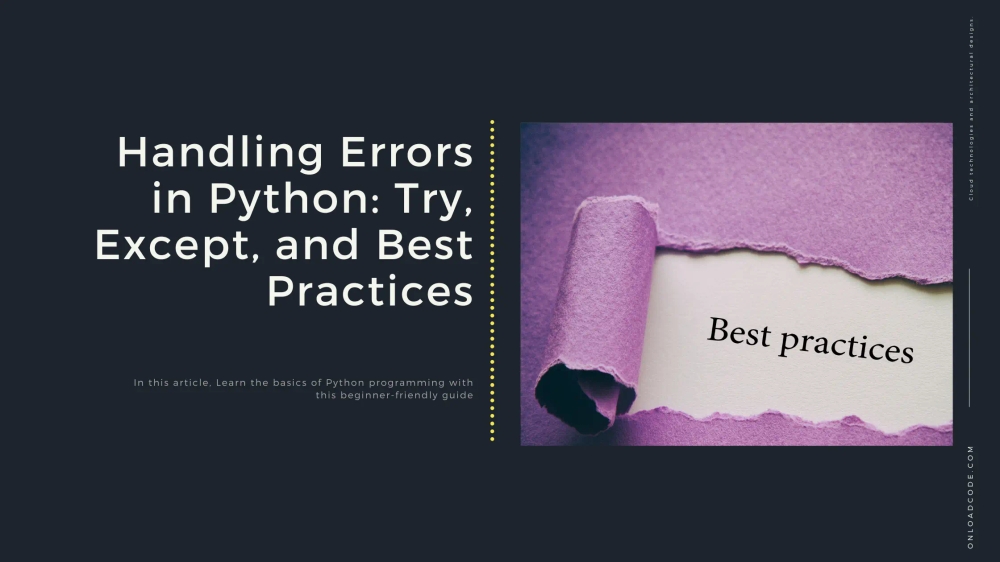
Handling Errors in Python: Try, Except, and Best Practices
Errors are an inevitable part of programming. Python provides the try-except
block to handle exceptions effectively, preventing programs from crashing unexpectedly.
Why Handle Errors?
- Prevents program crashes: Allows the program to continue running despite errors.
- Improves user experience: Provides meaningful error messages.
- Enhances debugging: Helps track and fix bugs efficiently.
Using Try-Except Blocks
A try
block is used to wrap code that might raise an exception. The except
block catches and handles the error.
try:
x = 10 / 0 # This will raise a ZeroDivisionError
except ZeroDivisionError:
print("Error: Cannot divide by zero!")
Handling Multiple Exceptions
You can handle multiple exceptions by specifying multiple except
blocks.
try:
num = int("abc") # This will raise a ValueError
except ZeroDivisionError:
print("Error: Division by zero!")
except ValueError:
print("Error: Invalid input!")
Using the Exception Object
You can capture exception details using as
.
try:
result = 10 / 0
except Exception as e:
print(f"An error occurred: {e}")
The Finally Block
The finally
block executes no matter what, making it useful for cleanup tasks.
try:
file = open("data.txt", "r")
except FileNotFoundError:
print("Error: File not found!")
finally:
print("Execution complete.")
Raising Exceptions
Use the raise
keyword to trigger exceptions manually.
def check_age(age):
if age < 18:
raise ValueError("Age must be 18 or older.")
print("Access granted.")
check_age(16) # Raises ValueError
Best Practices for Error Handling
- Be specific with exceptions: Catch only the exceptions you expect.
- Avoid bare
except
: Always specify an exception type. - Use logging instead of print: Helps in debugging.
- Close resources properly: Use
finally
orwith
statements.
Conclusion
Handling errors properly improves code reliability and user experience. Using try-except
correctly ensures your programs run smoothly even in unexpected situations.
Quiz Questions
- What is the purpose of a
try-except
block in Python? - How can you handle multiple exceptions in a single block?
- What does the
finally
block do in exception handling? - How do you manually raise an exception in Python?
- Why should you avoid using a bare
except
statement?