Published
- 2 min read
Getting Started with Python: A Beginner's Guide
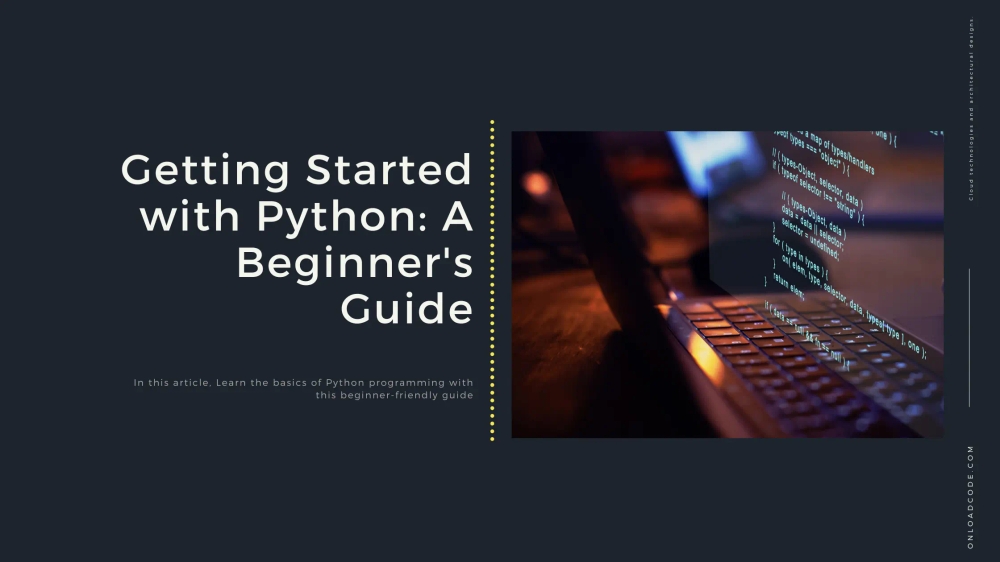
Getting Started with Python: A Beginner’s Guide
Python is one of the most popular and versatile programming languages. It’s known for its readability and simplicity, making it an excellent choice for beginners. This guide will help you take your first steps in learning Python.
Why Learn Python?
- Easy to Read and Write: Python syntax is clear and concise.
- Versatile Applications: Use Python for web development, data analysis, machine learning, automation, and more.
- Large Community: Access vast resources and community support.
Installing Python
- Download Python: Go to the official Python website and download the latest version for your operating system.
- Run the Installer:
- On Windows:
- Run the downloaded installer.
- Check the box that says “Add Python to PATH.”
- Click “Install Now.”
- Wait for the installation to complete and click “Close.”
- On Mac:
- Open the downloaded
.pkg
file. - Follow the installation steps and proceed with the default settings.
- Python is usually added to PATH automatically on Mac.
- Open the downloaded
- On Windows:
- Verify Installation: Open your terminal or command prompt and type:
python --version
You should see the installed Python version displayed.
Setting Up Your Environment
- Install an IDE or Text Editor: Popular options include VSCode, PyCharm, or Sublime Text.
- Create a Virtual Environment: This helps manage dependencies.
python -m venv myenv
- Activate the Virtual Environment:
- On Windows:
myenv\Scripts\activate
- On Mac/Linux:
source myenv/bin/activate
- Deactivate the Environment: When done, deactivate it by typing:
deactivate
Writing Your First Python Program
- Open a text editor (like VSCode, Sublime Text, or even Notepad).
- Type the following code:
print("Hello, Python!")
- Save the file as
hello.py
. - Open the terminal, navigate to the folder where you saved the file, and run:
python hello.py
You should see the output:
Hello, Python!
Understanding Basic Concepts
Variables
Variables store data values. Example:
name = "Alice"
print(name)
Data Types
Python has various data types like integers, floats, strings, and booleans:
age = 25 # Integer
height = 5.8 # Float
is_student = True # Boolean
Comments
Use #
to write comments in Python:
# This is a comment
print("This will run.")
Next Steps
- Practice: Try writing small programs.
- Explore Built-in Functions: Get familiar with functions like
len()
,type()
, andinput()
. - Learn Control Flow: Understand how to use
if
statements and loops.
Resources for Learning Python
Conclusion
Starting with Python is straightforward. With consistent practice, you’ll be able to build robust applications. Keep experimenting and enjoy your coding journey!