Published
- 3 min read
Functional Programming
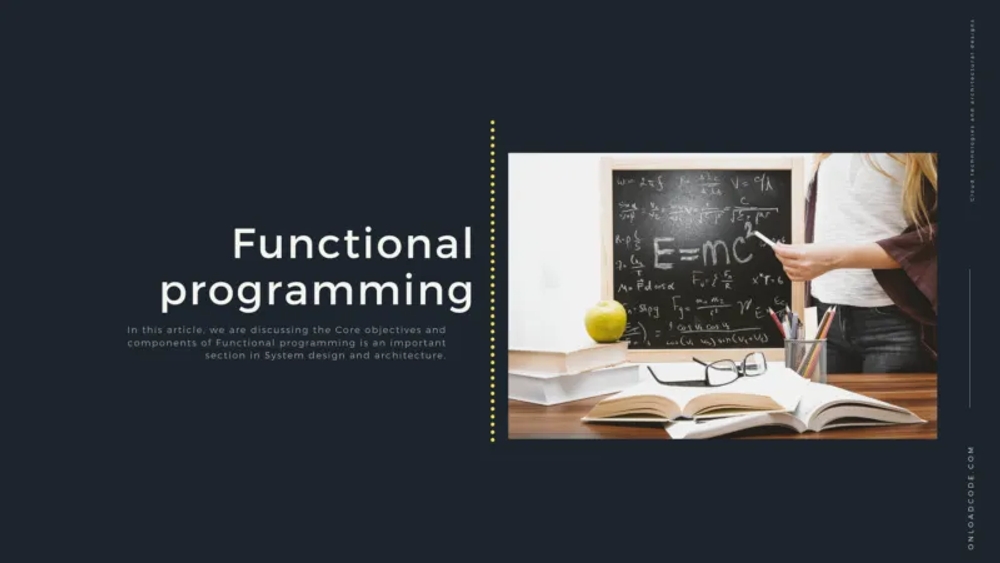
What is Functional Programming
Functional programming is a programming model in a structure and component building style of computer programs. It treats computation as an evaluation of mathematical functions and prevents variable-state and distorted data. This approach builds software with clean tasks, sharing partnerships, and avoiding side effects and mutable data. Unlike object-oriented programming, functional programming’s application state flows through clean functions without shared states.
Functional programming emphasizes principles like pure functions, immutability, and function composition, making it more concise, predictable, and easier to test than imperative or object-oriented code. However, its academic language and learning curve can make it challenging for beginners.
Characteristics of Functional Programming
- Designed around mathematical functions, using conditional expressions and iterations.
- Supports high-level tasks and lazy evaluation.
- Avoids language flow control structures like loops and relies on direct tasks and function calls.
- Supports concepts like abstraction, encapsulation, inheritance, and polymorphism.
Advantages of Functional Programming
- Error-Free Code: Avoids state changes and side effects.
- Parallel Programming: Tasks work in parallel efficiently.
- Efficiency: Independent units allow parallel execution.
- Nested Functions: Supports deep nesting.
- Lazy Evaluation: Constructs like lazy lists and maps are supported.
- Functional programming is suitable for tasks involving multiple operations on the same data set and artificial intelligence applications like machine learning and language processing.
Key Concepts
Pure Functions
A pure function always produces the same output for the same input without side effects. This guarantees reference transparency and ensures predictable behavior.
Function Composition
Combining two or more functions to create a new function or perform calculations. For example, f(g(x))
in JavaScript represents function composition.
Shared State
Shared state refers to variables or memory shared between domains. Functional programming avoids shared states, relying on immutable data structures to simplify changes and enhance code reliability.
Immutability
Immutable objects cannot be changed after creation. Functional programming heavily relies on immutable data to prevent unexpected state changes and ensure consistent behavior.
In JavaScript, constants (const
) bind names to variables but do not create immutable objects. Functional programming languages often use persistent data structures to ensure true immutability.
Side Effects
A side effect is any observable change outside a function’s return value. Examples include:
- Modifying external variables or objects.
- Writing to the console, files, or networks.
- Triggering external processes.
Functional programming minimizes side effects, making code easier to understand and test.
Conclusion
Functional programming offers a robust framework for building scalable and efficient software. By adhering to principles like pure functions, immutability, and avoiding shared states, developers can create reliable and maintainable applications.
Thanks for reading the article Functional Programming as an essential component in System Design and Architecture.
Originally published at https://onloadcode.com on January 3, 2021.