Published
- 2 min read
File Handling in Python: Reading and Writing Files Simplified
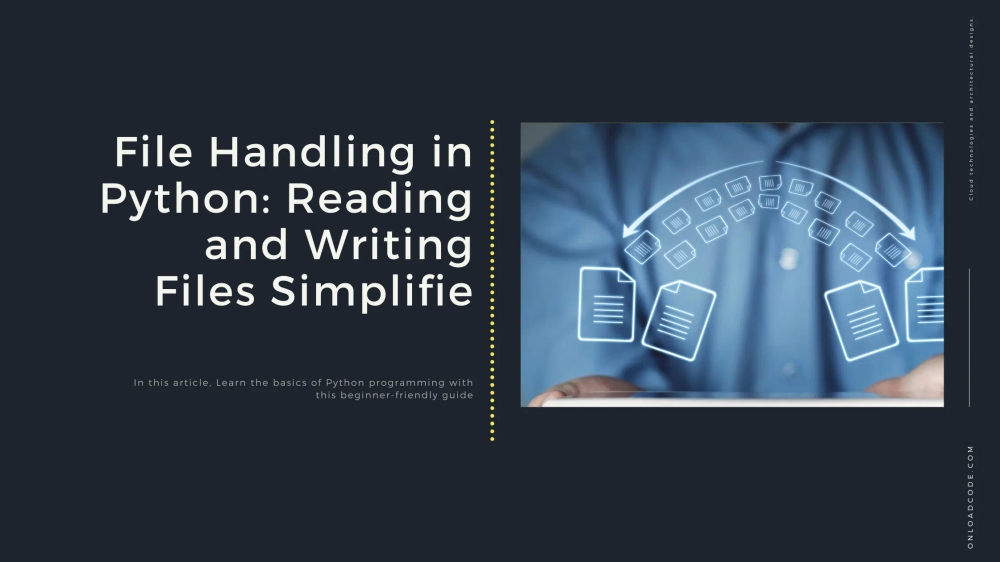
File Handling in Python: Reading and Writing Files Simplified
Working with files is an essential part of programming. Python provides simple and efficient ways to read, write, and manipulate files.
Why File Handling is Important
- Data Storage: Save information for future use.
- Processing Large Data: Work with large files efficiently.
- Automation: Read and write logs, configurations, and reports.
Opening a File in Python
Python’s built-in open()
function is used to open files.
file = open("example.txt", "r") # Open file in read mode
File Modes
Mode | Description |
---|---|
r | Read (default) |
w | Write (overwrites file) |
a | Append (adds to file) |
x | Create new file |
b | Binary mode |
t | Text mode (default) |
Reading Files
To read a file, use the read()
or readline()
methods.
with open("example.txt", "r") as file:
content = file.read()
print(content) # Prints file content
Reading Line by Line
with open("example.txt", "r") as file:
for line in file:
print(line.strip())
Writing to Files
To write data to a file, use the write()
method.
with open("example.txt", "w") as file:
file.write("Hello, Python!")
Appending to a File
with open("example.txt", "a") as file:
file.write("Adding more content.\n")
Working with Binary Files
Use rb
or wb
mode to handle binary files like images.
with open("image.png", "rb") as file:
data = file.read()
Closing Files Properly
Always close files after use, or use with open()
to handle it automatically.
file = open("example.txt", "r")
print(file.read())
file.close()
Best Practices for File Handling
- Use
with open()
: Ensures proper closing of files. - Handle exceptions: Use
try-except
to catch errors. - Avoid overwriting files accidentally: Check before writing.
Conclusion
Python makes file handling simple and efficient. Understanding how to read, write, and manage files is crucial for real-world applications.
Quiz Questions
- What function is used to open a file in Python?
- What is the difference between
r
,w
, anda
modes? - How do you read a file line by line?
- What does
with open()
do in file handling? - How can you handle errors when working with files?