Published
- 2 min read
An Introduction to Object-Oriented Programming in Python
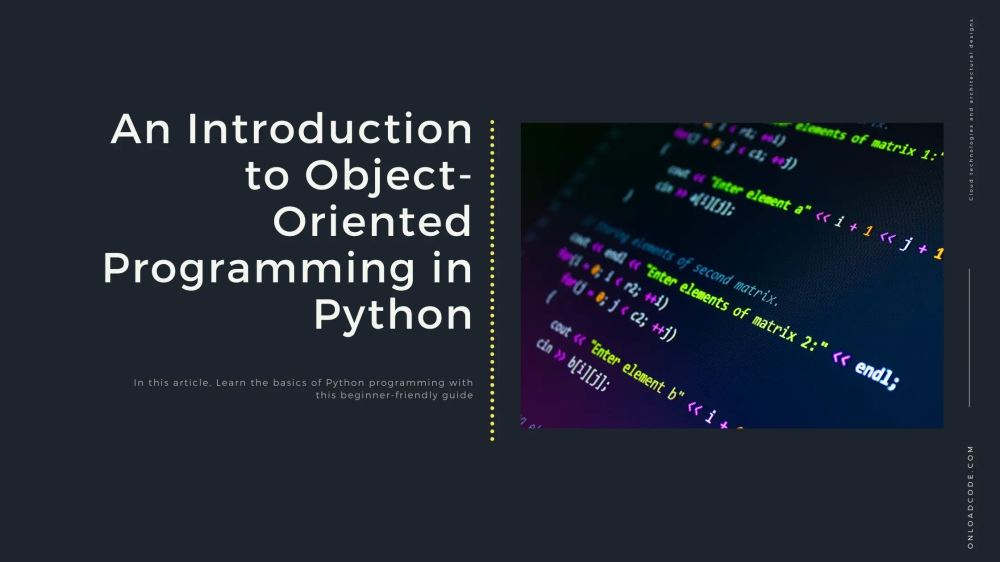
An Introduction to Object-Oriented Programming in Python
Object-Oriented Programming (OOP) is a programming paradigm that uses objects and classes to structure code efficiently. Python, being an object-oriented language, provides powerful features to implement OOP principles.
Why Use Object-Oriented Programming?
- Modularity: Code is organized into reusable structures.
- Encapsulation: Data is bundled with functions that operate on that data.
- Inheritance: New classes can derive from existing ones, reducing redundancy.
- Polymorphism: Objects of different classes can be treated as objects of a common superclass.
Defining a Class in Python
A class is a blueprint for creating objects. Use the class
keyword to define one.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
Creating Objects
An object is an instance of a class.
p1 = Person("Alice", 30)
p1.greet() # Output: Hello, my name is Alice and I am 30 years old.
Encapsulation
Encapsulation restricts direct access to variables and allows controlled modification.
class BankAccount:
def __init__(self, balance):
self.__balance = balance # Private variable
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance
account = BankAccount(1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
Inheritance
Inheritance allows one class to acquire the properties of another.
class Animal:
def speak(self):
print("This animal makes a sound.")
class Dog(Animal):
def speak(self):
print("Woof Woof!")
d = Dog()
d.speak() # Output: Woof Woof!
Polymorphism
Polymorphism enables different classes to use the same method name.
class Cat:
def speak(self):
print("Meow!")
def make_animal_speak(animal):
animal.speak()
c = Cat()
d = Dog()
make_animal_speak(c) # Output: Meow!
make_animal_speak(d) # Output: Woof Woof!
Conclusion
Understanding OOP in Python is crucial for writing scalable and maintainable applications. Practice creating classes, objects, and implementing OOP concepts to improve your programming skills.
Quiz Questions
- What is a class in Python?
- How do you create an object in Python?
- What is encapsulation, and why is it important?
- How does inheritance work in Python?
- What is polymorphism in object-oriented programming?